Since I’ve a YouTube channel, I like to see how it’s going. So I was constantly going to YouTube Studio to check my analytics, but… this is very repetitive and I don’t really care about the more in-depth statistics. So I started thinking about another way…
What if I could display live YouTube data on my LCD screen using an ESP32?
After some searching, I found the YouTube Data API, which has the ability to fetch live YouTube channel data with an ESP32. So, “I” (ChatGPT did the most of the work) programmed a simple program and I’m sharing it with the world.
Required hardware:
We can’t build the coolest project with air yet (sad oooh), so you’ll need the necessary components:
- I2C LCD 20×4 (or 16×2, but you’ll need some easy changes to the code)
- ESP32
- Cables
- Requirements to upload the code
0: Wiring the LCD
For this project, I wired the LCD screen to the ESP32 as follows:
LCD (I2C) | ESP32 |
---|---|
VCC | 3.3V/5V |
GND | GND |
SDA | GPIO 21 |
SCL | GPIO 22 |
Please note that each ESP32 or LCD may have a different pinout, so do your research!
1. YouTube Data API
To use the YouTube Data API, we need permission with an API key. Don’t worry, it’s free and you get the key right away. Go to Google Cloud Console and create or use an existing project.
After the creation of your project, you should be greeted with this screen:
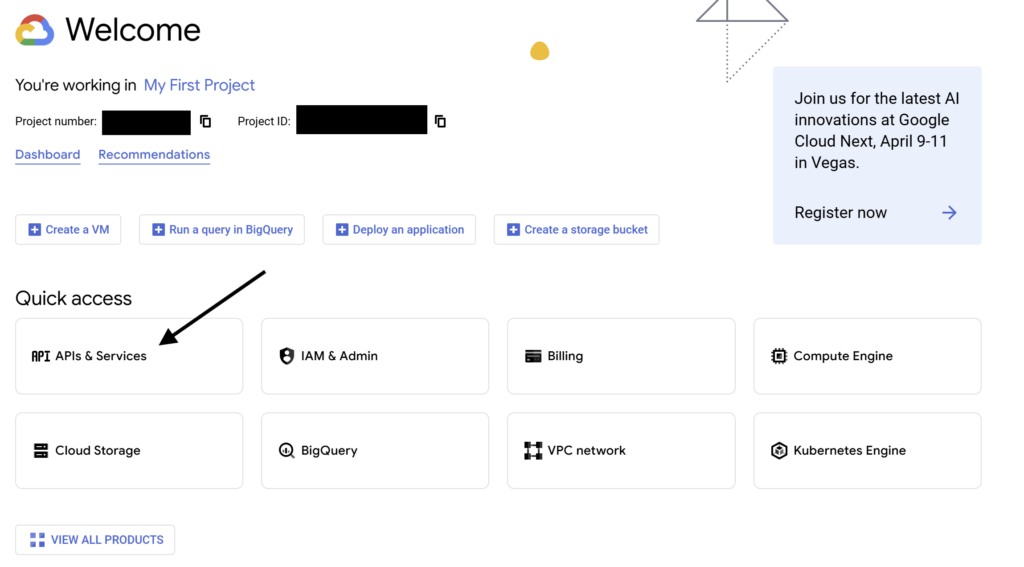
Click on “API & Services”, then on the blue rectangle that says “Enable APIs and Services” and search for “YouTube Data API v3”. Next, select the result and click on the blue rectangle “enable”. Next, select the result and click on the blue rectangle “enable”.
You will be redirected to a new page where you can click on the grey rectangle “Create Credentials”. We’re now prompted with some questions:
- Select “Public data”
- Copy and save your API key
2: Channel ID
Next, we need to get the Channel ID of the YouTube channel we want to monitor. This can be found in YouTube Studio at the URL /channel/UC…
My channel URL is:
https://www.youtube.com/channel/UCf2vflWUFGHJMhZlGNxYO9Q
With the Channel ID:
UCf2vflWUFGHJMhZlGNxYO9Q
3: Code
Libraries:
Time to code! First, make sure you have the right libraries installed in Arduino IDE:
- Wire.h (should be automatically installed)
- LiquidCrystal_I2C.h by Frank de Brabander
- Wifi.h by Arduino
- HTTPClient.h by Adrian McEwen
- ArduinoJSON by Benoit Blanchon
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
#include <WiFi.h>
#include <HTTPClient.h>
#include <ArduinoJson.h>
// WiFi credentials
const char* ssid = "Your_SSID";
const char* password = "Your_PASSWORD";
// YouTube API details
const char* API_KEY = "YOUR_YOUTUBE_API_KEY";
const char* CHANNEL_ID = "YOUR_CHANNEL_ID";
// LCD setup (I2C address 0x27, 20 cols, 4 rows)
//Change this if you use a different display.
LiquidCrystal_I2C lcd(0x27, 20, 4);
StaticJsonDocument<1024> getJsonYoutubePayload() {
String url = "https://www.googleapis.com/youtube/v3/channels?part=statistics&id=" + String(CHANNEL_ID) + "&key=" + String(API_KEY);
HTTPClient http;
http.begin(url);
int httpCode = http.GET();
StaticJsonDocument<1024> doc;
if (httpCode > 0) {
String payload = http.getString();
Serial.println("API Response: " + payload); // Debugging output
DeserializationError error = deserializeJson(doc, payload);
if (error) {
Serial.println("JSON Parsing Error: " + String(error.c_str()));
}
} else {
Serial.println("HTTP Error: " + String(httpCode));
}
http.end(); // Free up resources
return doc; // Return JSON document
}
int getSubscriberCount(StaticJsonDocument<1024>& doc) {
if (!doc.containsKey("items") || doc["items"].size() == 0) {
Serial.println("Error: 'items' array is missing!");
return -1;
}
return doc["items"][0]["statistics"]["subscriberCount"].as<String>().toInt();
}
// Function to extract total view count
int getViewCount(StaticJsonDocument<1024>& doc) {
if (!doc.containsKey("items") || doc["items"].size() == 0) {
return -1;
}
return doc["items"][0]["statistics"]["viewCount"].as<String>().toInt();
}
// Function to extract total uploads
int getUploads(StaticJsonDocument<1024>& doc) {
if (!doc.containsKey("items") || doc["items"].size() == 0) {
return -1;
}
return doc["items"][0]["statistics"]["videoCount"].as<String>().toInt();
}
void setup() {
Serial.begin(115200);
lcd.init();
lcd.backlight();
// Connect to WiFi
WiFi.begin(ssid, password);
lcd.setCursor(0, 0);
lcd.print("Connecting to WiFi");
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("WiFi Connected!");
}
void loop() {
StaticJsonDocument<1024> youtubePayload = getJsonYoutubePayload();
int subs = getSubscriberCount(youtubePayload);
int totalViews = getViewCount(youtubePayload);
int uploads = getUploads(youtubePayload);
if (subs != -1) {
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Subs: " + String(subs));
lcd.setCursor(0, 1);
lcd.print("Total views: " + String(totalViews));
lcd.setCursor(0, 2);
lcd.print("Uploads: " + String(uploads));
} else {
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("API Error!");
}
delay(60000); // Update every 60 seconds
}
How it works:
- Connects ESP32 to the WiFi
- Get the JSON payload to use in other functions
- Prints API response to Serial monitor (Baud: 115200)
- Store results to variables to be displayed on LCD
- Updates every 60s
Conclusion
Sending an API request with an ESP32 to retrieve general YouTube Channel info is a fun and an interesting project. I hope you enjoyed!
Goodbye!
Leave a Reply