Loops in Visual Bukkit 6 (and in general) are my best friends… because I have to write less code! In Visual Bukkit, loops are cleanly divided into five main types, each with its own use case. If you prefer videos over text, I’ve got you covered—watch my walkthrough here:
1: The Number Loop
This loop runs a set number of times and keeps track of the count in a variable called Loop Number
.
In this example, the loop will display 0 to 4 to the Command Sender
, because a loop always starts with 0.
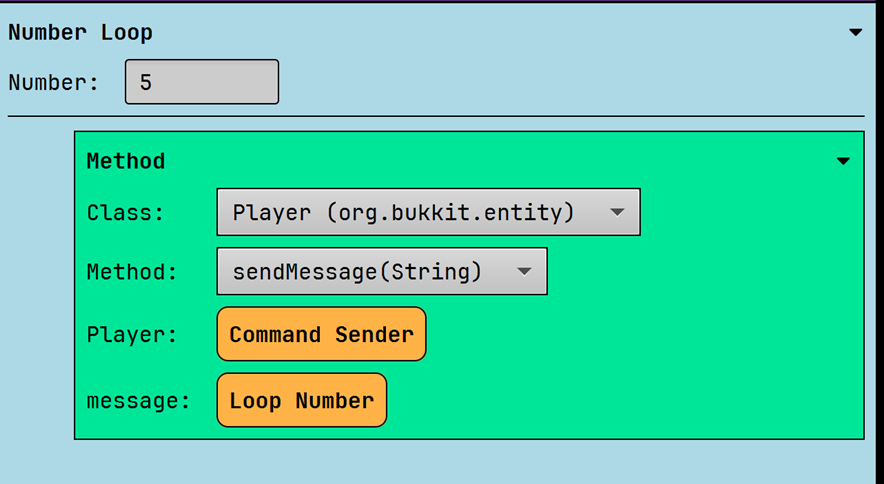
In my video, I used the loop to spawn in 5 fireworks.
2: The Advanced Number Loop
The Number Loop
also has a big brother, The Advanced Number Loop
. As the name suggests, it’s a little more advanced:
- Start: The value to begin from.
- End: The value where the count should stop
- Update Value: How much to increase or decrease the
start value
per loop. - Comparison/Condition: Compares the
start value
to the changedend value
. If it’s true, it keeps looping.
It only works with integers, so a 1.8 automatically becomes 1!
The Number Loop
variable also works here. In this case, the result is from 1 to 5. (1<6, 2<6,…5<6)
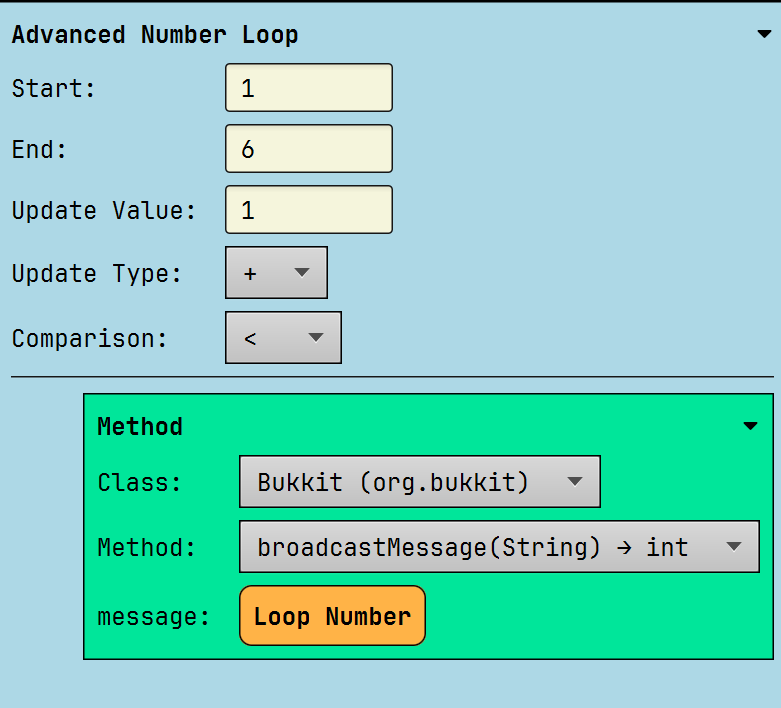
3: While Loop
This one keeps looping as long as a condition is true. That’s powerful—but potentially risky if you forget to break the loop.
Tip: Use the Negated mode
to flip true to false (and vice versa). This is super handy when checking if something isn’t something.
In this example, the loop goes from 1.0 to 10.0.
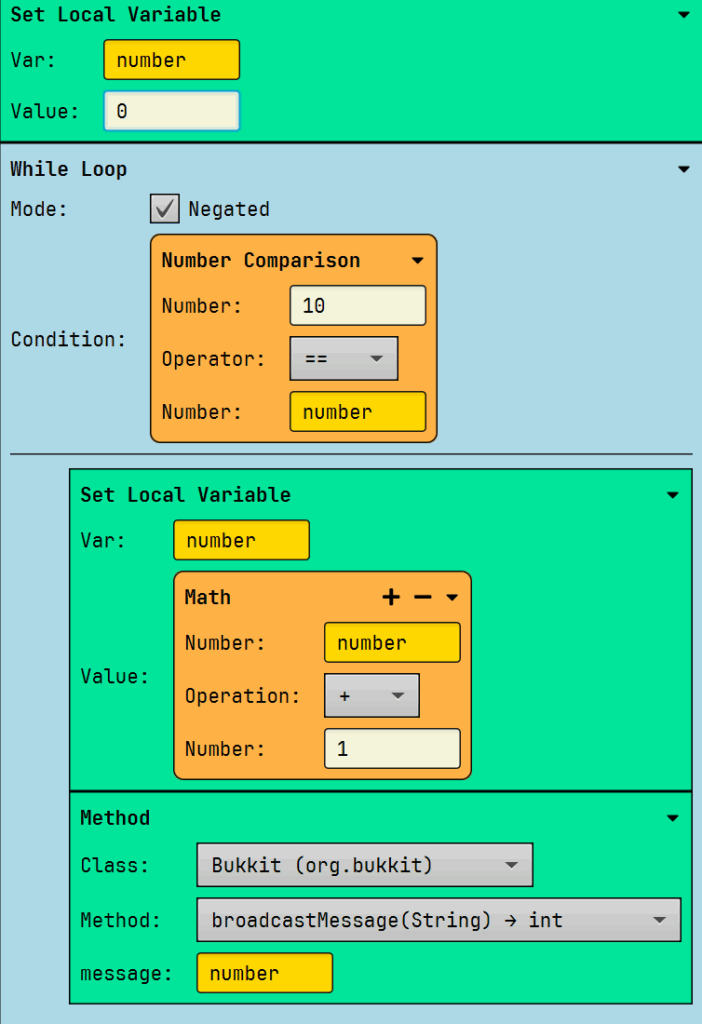
4: The List Loop
Instead of looping a set number of times, it loops through each item of the list and stores it in the Loop Value. This is very handy if you want to send e.g a title to all the online players:
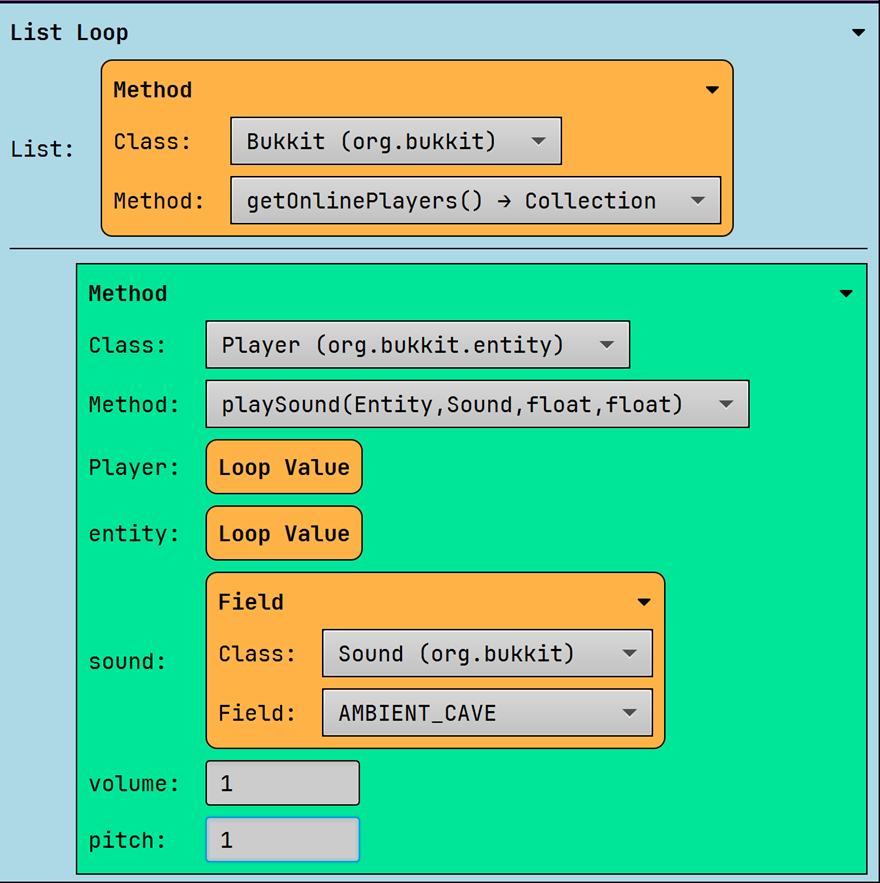
All the previous loops could be stopped with Break loop
. The Continue Loop
skips an iteration of a loop, so it doesn’t start a loop back up after a break loop. I haven’t explained this well in my video…
5: The Schedule Task
The Schedule Task
isn’t directly a loop. It’s from the Bukkit API, meant for scheduling a task (duh) and looping in repetitive mode.
Both of these option can be done with Asynchronous
and Synchronous
execution.
Synchronous and Asynchronous?
Synchronous: Imagine a single-file line at a McDonalds. Each person (task) is served one at a time. The next person can’t be helped until the current person is finished. In Spigot, synchronous tasks run on the main server thread, one after another.
Asynchronous: Now, picture multiple checkout lanes. Several people (tasks) can be served simultaneously. In Spigot, asynchronous tasks run on separate threads, independent of the main server thread.
Another analogy: Think of the main server thread as the conductor of an orchestra. If the conductor stops, the music stops. Asynchronous tasks are like extra musicians practicing in separate rooms. They can work independently without interrupting the main performance.
When to use what?
Use synchronous tasks for anything involving the Bukkit/Minecraft API.
Use asynchronous tasks for external stuff, like database queries.
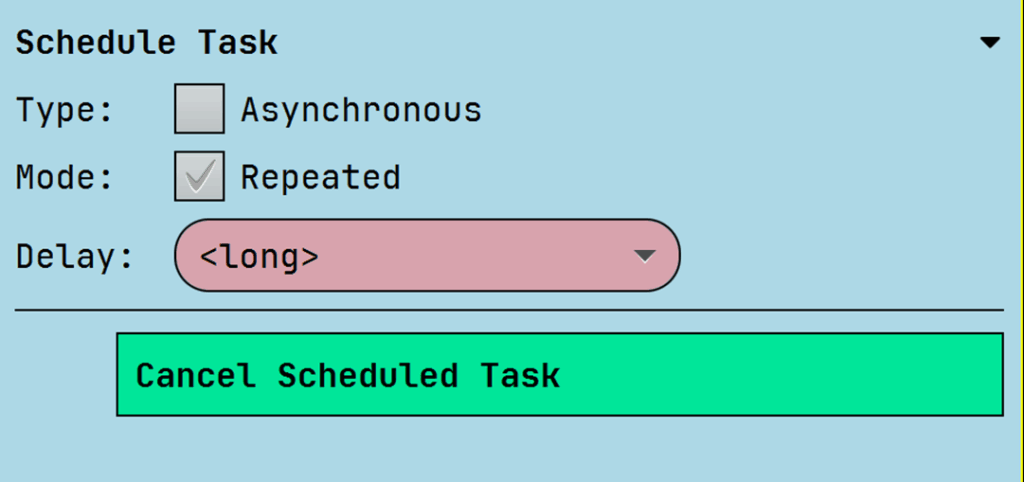
To stop a Schedule Task
, use Cancel Schedule Task
.
Leave a Reply